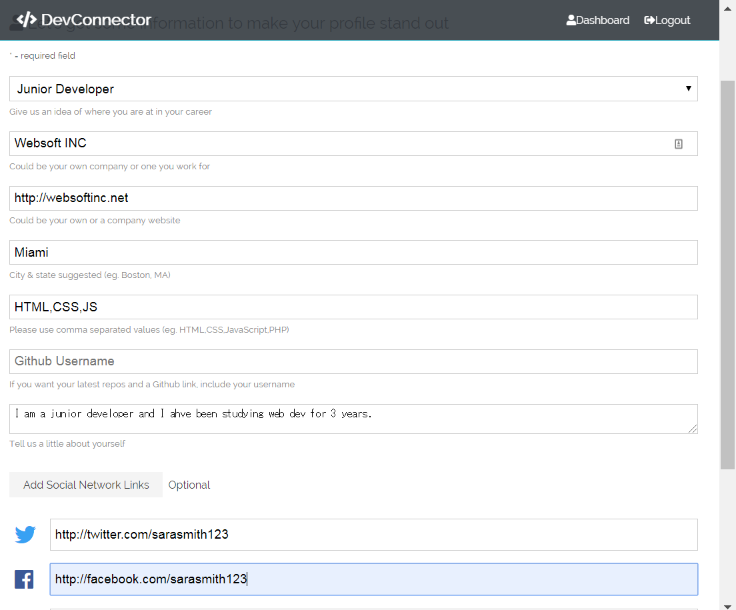
今回は、プロフィール部分のactionを作成しました。新しく学んだことは、import { withRouter} from 'react-router-dom'; を使って、history、そして、history.pushを使って別のページへリダイレクトするように設定することです。
①client/src/actions/profile.jsにプロフィールの作成orアップデートのコードを書き加える。history.pushメソッドを初めて使用。
元々あったactions/profile.jsに以下のコードを書き加えました。コードの最後の方で、 if (!edit) { history.push("/dashboard");というコードを使って、ダッシュボードにリダイレクトされるように設定しています。
また、<a href= ></a>で囲っていた部分も<Link to="/ "></Link>に書き換えています。
// Create or update profile export const createProfile = ( formData, history, edit = false ) => async dispatch => { try { const config = { headers: { "Content-Type": "application/json" } }; const res = await axios.post("/api/profile", formData, config); dispatch({ type: GET_PROFILE, payload: res.data }); dispatch(setAlert(edit ? "Profile Updated" : "Profile Created", "success")); if (!edit) { history.push("/dashboard"); } } catch (err) { const errors = err.response.data.errors; if (errors) { errors.forEach(error => dispatch(setAlert(error.msg, "danger"))); } dispatch({ type: PROFILE_ERROR, payload: { msg: err.response.statusText, status: err.response.status } }); } };
②client/src/components/profile-form/CreateProfile.jsにwithRouterをインポートしてhistoryが使えるようにする。
少々長いのですが、書き換えた(書き加えた)コードは、最初のimport部分でimport { withRouter} from 'react-router-dom'; として、コード中程で何度かhistoryを使用している部分です。また、最後のconnect部分にもwithRouterを書き加えて使用できるようにしました。
import React, { useState, Fragment } from "react"; import { Link, withRouter } from "react-router-dom"; import PropTypes from "prop-types"; import { connect } from "react-redux"; import { createProfile } from "../../actions/profile"; const CreateProfile = ({ createProfile, history }) => { const [formData, setFormData] = useState({ company: "", website: "", location: "", status: "", skills: "", githubusername: "", bio: "", twitter: "", facebook: "", linkedin: "", youtube: "", instagram: "" }); const [displaySocialImputs, toggleSocialImputs] = useState(false); const { company, website, location, status, skills, githubusername, bio, twitter, facebook, linkedin, youtube, instagram } = formData; const onChange = e => setFormData({ ...formData, [e.target.name]: e.target.value }); const onSubmit = e => { e.preventDefault(); createProfile(formData, history, true); }; return ( <Fragment> <h1 className='large text-primary'>Create Your Profile</h1> <p className='lead'> <i className='fas fa-user' /> Let's get some information to make your profile stand out </p> <small>* = required field</small> <form className='form' onSubmit={e => onSubmit(e)}> <div className='form-group'> <select name='status' value={status} onChange={e => onChange(e)}> <option value='0'>* Select Professional Status</option> <option value='Developer'>Developer</option> <option value='Junior Developer'>Junior Developer</option> <option value='Senior Developer'>Senior Developer</option> <option value='Manager'>Manager</option> <option value='Student or Learning'>Student or Learning</option> <option value='Instructor'>Instructor or Teacher</option> <option value='Intern'>Intern</option> <option value='Other'>Other</option> </select> <small className='form-text'> Give us an idea of where you are at in your career </small> </div> <div className='form-group'> <input type='text' placeholder='Company' name='company' value={company} onChange={e => onChange(e)} /> <small className='form-text'> Could be your own company or one you work for </small> </div> <div className='form-group'> <input type='text' placeholder='Website' name='website' value={website} onChange={e => onChange(e)} /> <small className='form-text'> Could be your own or a company website </small> </div> <div className='form-group'> <input type='text' placeholder='Location' name='location' value={location} onChange={e => onChange(e)} /> <small className='form-text'> City & state suggested (eg. Boston, MA) </small> </div> <div className='form-group'> <input type='text' placeholder='* Skills' name='skills' value={skills} onChange={e => onChange(e)} /> <small className='form-text'> Please use comma separated values (eg. HTML,CSS,JavaScript,PHP) </small> </div> <div className='form-group'> <input type='text' placeholder='Github Username' name='githubusername' value={githubusername} onChange={e => onChange(e)} /> <small className='form-text'> If you want your latest repos and a Github link, include your username </small> </div> <div className='form-group'> <textarea placeholder='A short bio of yourself' name='bio' value={bio} onChange={e => onChange(e)} /> <small className='form-text'>Tell us a little about yourself</small> </div> <div className='my-2'> <button onClick={() => toggleSocialImputs(!displaySocialImputs)} type='button' className='btn btn-light' > Add Social Network Links </button> <span>Optional</span> </div> {displaySocialImputs && ( <Fragment> <div className='form-group social-input'> <i className='fab fa-twitter fa-2x' /> <input type='text' placeholder='Twitter URL' name='twitter' value={twitter} onChange={e => onChange(e)} /> </div> <div className='form-group social-input'> <i className='fab fa-facebook fa-2x' /> <input type='text' placeholder='Facebook URL' name='facebook' value={facebook} onChange={e => onChange(e)} /> </div> <div className='form-group social-input'> <i className='fab fa-youtube fa-2x' /> <input type='text' placeholder='YouTube URL' name='youtube' value={youtube} onChange={e => onChange(e)} /> </div> <div className='form-group social-input'> <i className='fab fa-linkedin fa-2x' /> <input type='text' placeholder='Linkedin URL' name='linkedin' value={linkedin} onChange={e => onChange(e)} /> </div> <div className='form-group social-input'> <i className='fab fa-instagram fa-2x' /> <input type='text' placeholder='Instagram URL' name='instagram' value={instagram} onChange={e => onChange(e)} /> </div> </Fragment> )} <input type='submit' className='btn btn-primary my-1' /> <Link className='btn btn-light my-1' to='/dashboard'> Go Back </Link> </form> </Fragment> ); }; CreateProfile.propTypes = { createProfile: PropTypes.func.isRequired }; export default connect( null, { createProfile } )(withRouter(CreateProfile));
React RouterでwithRouterを使用して、history.pushでリダイレクトを行う方法については、Qiitaの@junaraさんの記事も読んで学習しました。
⇒react-routerのページ遷移をhandleで行う時にはwithRouterを使う
現在使用している教材と学習時間:本日の学習時間2.5時間
Udemy:MERN Stack Front To Back: Full Stack React, Redux & Node.js by Brad Traversy
★Section9:Dashboard & Profile Management
-Lec 48 Create Profile Action (このブログ記事に書いた内容)
-Lec 49 Edit Profile (本日はここまで完了)
進捗状況:68%
学習時間2.5時間
~本日は休みにしている教材~
Udemy:The Complete Web Developer: Zero to Mastery by Andrei Neagoie
進捗状況: 92%
★参考にした本★
「React.js & Next.js超入門」掌田津耶乃 (秀和システム)
Section4-1: Reduxを使ってみよう
★参照記事★
Quiita参照記事⇒react-routerのページ遷移をhandleで行う時にはwithRouterを使う