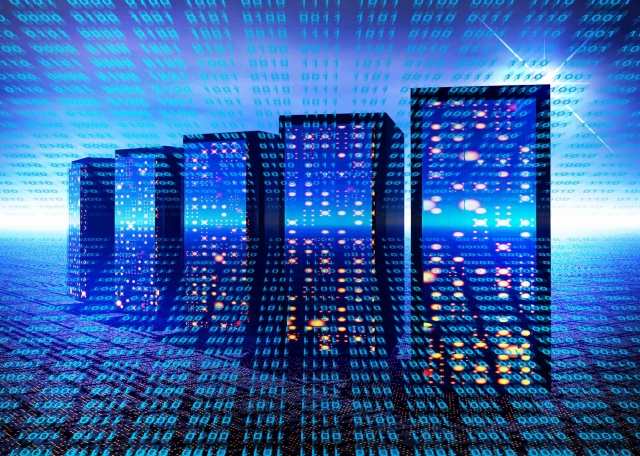
引き続きroutesを作成しました。今回は、ユーザーとプロフィールの削除、ユーザーのprofileの中の経験と学歴を追加・削除するroutesを作成しました。
今回書いたroutesのコード(経験・学歴のところはコピペで対応)
// @route DELETE api/profile
// @desc Delete profile, user & posts
// @access Private
router.delete("/", auth, async (req, res) => {
try {
// @todo - remove users posts
// Remove profile
await Profile.findOneAndRemove({ user: req.user.id });
// Remove user
await User.findOneAndRemove({ _id: req.user.id });
res.json({ ums: "User deleted" });
} catch (err) {
console.error(err.message);
res.status(500).send("Server Error");
}
});
// @route PUT api/experience
// @desc Add profile experience
// @access Private
router.put(
"/experience",
[
auth,
[
check("title", "Title is required")
.not()
.isEmpty(),
check("company", "Company is required")
.not()
.isEmpty(),
check("from", "From date is required")
.not()
.isEmpty()
]
],
async (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const {
title,
company,
location,
from,
to,
current,
description
} = req.body;
const newExp = {
title,
company,
location,
from,
to,
current,
description
};
try {
const profile = await Profile.findOne({ user: req.user.id });
profile.experience.unshift(newExp);
await profile.save();
res.json(profile);
} catch (err) {
console.error(err.message);
res.status(500).send("Server Error");
}
}
);
// @route DELETE api/experience/:exp_id
// @desc Delete experience from profile
// @access Private
router.delete("/experience/:exp_id", auth, async (req, res) => {
try {
const profile = await Profile.findOne({ _id: req.user.id });
// Get remove index
const removeIndex = profile.experience
.map(item => item.id)
.indexOf(req.params.exp_id);
profile.experience.splice(removeIndex, 1);
await profile.save();
res.json(profile);
} catch (err) {
console.error(err.message);
res.status(500).send("Server Error");
}
});
//lec22
// @route PUT api/education
// @desc Add profile education
// @access Private
router.put(
"/education",
[
auth,
[
check("school", "Title is required")
.not()
.isEmpty(),
check("degree", "Degree is required")
.not()
.isEmpty(),
check("fieldofstudy", "Field of study is required")
.not()
.isEmpty(),
check("from", "From date is required")
.not()
.isEmpty()
]
],
async (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const {
school,
degree,
fieldofstudy,
from,
to,
current,
description
} = req.body;
const newEdu = {
school,
degree,
fieldofstudy,
from,
to,
current,
description
};
try {
const profile = await Profile.findOne({ user: req.user.id });
profile.education.unshift(newEdu);
await profile.save();
res.json(profile);
} catch (err) {
console.error(err.message);
res.status(500).send("Server Error");
}
}
);
// @route DELETE api/education/:edu_id
// @desc Delete education from profile
// @access Private
router.delete("/education/:edu_id", auth, async (req, res) => {
try {
const profile = await Profile.findOne({ _id: req.user.id });
// Get remove index
const removeIndex = profile.education
.map(item => item.id)
.indexOf(req.params.edu_id);
profile.education.splice(removeIndex, 1);
await profile.save();
res.json(profile);
} catch (err) {
console.error(err.message);
res.status(500).send("Server Error");
}
});
最後は、
module.exports = router;
で、これは最初からずっと同じです。
次回の課題はPOSTMANの設定内容修正
本日も一部POSTMANの動きがおかしかったのですが、おそらく私が前回操作した時にJWT(Json Web Tokens)で取得したユーザーのtokenをしっかりセーブしておかなかったからだと思います。
また、色々と実験的に自分でデータを追加したりしていたのも講座内容に沿っていないので削除予定です。今回は、間違いのないコードを書くことができたので、次回はPOSTMANでの設定のチェックです。
現在使用している教材と現在の状況:学習時間2.5時間
Udemy:MERN Stack Front To Back: Full Stack React, Redux & Node.js by Brad Traversy
Sec4: Profile API Routes
-Lec19 Delete Profile& User
-Lec20 Add Profile Experience
-Lec21 Delete Profile Experience
-Lec22 Add & Delete Profile Education
進捗状況:30%
学習時間2.5時間
~本日は休みにしている教材~
Udemy:The Complete Web Developer: Zero to Mastery by Andrei Neagoie
進捗状況: 92%
-参考にしたページ-
「express実践入門」小川充 (GitHub) (こちらのページについては次の記事に詳しく書きました。)